300
|
How do I get the handle of the cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->PutCellBold(vtMissing,var_Items->GetItemCell(h,long(0)),VARIANT_TRUE);
|
299
|
How do I retrieve the focused item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->PutItemBold(var_Items->GetFocusItem(),VARIANT_TRUE);
|
298
|
How do I get the number or count of child items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->AddItem(var_Items->GetChildCount(h));
|
297
|
How do I enumerate the visible items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->PutItemBold(var_Items->GetFirstVisibleItem(),VARIANT_TRUE);
var_Items->PutItemBold(var_Items->GetNextVisibleItem(var_Items->GetFirstVisibleItem()),VARIANT_TRUE);
|
296
|
How do I enumerate the siblings items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->PutItemBold(var_Items->GetNextSiblingItem(var_Items->GetFirstVisibleItem()),VARIANT_TRUE);
var_Items->PutItemBold(var_Items->GetPrevSiblingItem(var_Items->GetNextSiblingItem(var_Items->GetFirstVisibleItem())),VARIANT_TRUE);
|
295
|
How do I get the parent item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->PutItemBold(var_Items->GetItemParent(var_Items->GetItemChild(h)),VARIANT_TRUE);
|
294
|
How do I get the first child item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->PutItemBold(var_Items->GetItemChild(h),VARIANT_TRUE);
|
293
|
How do I enumerate the root items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutItemBold(var_Items->GetRootItem(0),VARIANT_TRUE);
var_Items->PutItemUnderline(var_Items->GetRootItem(1),VARIANT_TRUE);
|
292
|
I have a hierarchy, how can I count the number of root items
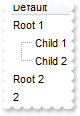
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root 1");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->AddItem("Root 2");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->AddItem(var_Items->GetRootCount());
|
291
|
How can I make an item unselectable, or not selectable
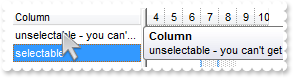
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Column");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("unselectable - you can't get selected");
var_Items->PutSelectableItem(h,VARIANT_FALSE);
var_Items->AddItem("selectable");
|
290
|
How can I hide or show an item
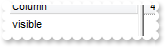
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Column");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("hidden");
var_Items->PutItemHeight(h,0);
var_Items->PutSelectableItem(h,VARIANT_FALSE);
var_Items->AddItem("visible");
|
289
|
How can I change the height for all items
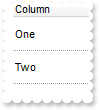
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutDefaultItemHeight(32);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem("One");
spGantt1->GetItems()->AddItem("Two");
|
288
|
How do I change the height of an item
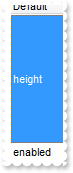
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutScrollBySingleLine(VARIANT_TRUE);
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemHeight(var_Items->AddItem("height"),128);
spGantt1->GetItems()->AddItem("enabled");
|
287
|
How do I disable or enable an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutEnableItem(var_Items->AddItem("disabled"),VARIANT_FALSE);
spGantt1->GetItems()->AddItem("enabled");
|
286
|
How do I display as strikeout a cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellStrikeOut(var_Items->AddItem("strikeout"),long(0),VARIANT_TRUE);
|
285
|
How do I display as strikeout a cell or an item
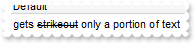
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("gets <s>strikeout</s> only a portion of text"),long(0),EXGANTTLib::exHTML);
|
284
|
How do I display as strikeout an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemStrikeOut(var_Items->AddItem("strikeout"),VARIANT_TRUE);
|
283
|
How do I underline a cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellUnderline(var_Items->AddItem("underline"),long(0),VARIANT_TRUE);
|
282
|
How do I underline a cell or an item
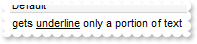
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("gets <u>underline</u> only a portion of text"),long(0),EXGANTTLib::exHTML);
|
281
|
How do I underline an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemUnderline(var_Items->AddItem("underline"),VARIANT_TRUE);
|
280
|
How do I display as italic a cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellItalic(var_Items->AddItem("italic"),long(0),VARIANT_TRUE);
|
279
|
How do I display as italic a cell or an item
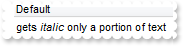
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("gets <i>italic</i> only a portion of text"),long(0),EXGANTTLib::exHTML);
|
278
|
How do I display as italic an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemItalic(var_Items->AddItem("italic"),VARIANT_TRUE);
|
277
|
How do I bold a cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellBold(var_Items->AddItem("bold"),long(0),VARIANT_TRUE);
|
276
|
How do I bold a cell or an item
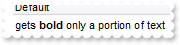
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("gets <b>bold</b> only a portion of text"),long(0),EXGANTTLib::exHTML);
|
275
|
How do I bold an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemBold(var_Items->AddItem("bold"),VARIANT_TRUE);
|
274
|
How do I change the foreground color for the item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
long hC = var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutItemForeColor(hC,RGB(255,0,0));
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
273
|
How do I change the visual appearance for the item, using your EBN technology
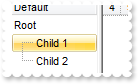
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetVisualAppearance()->Add(1,"c:\\exontrol\\images\\normal.ebn");
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
long hC = var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutItemBackColor(hC,0x1000000);
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
272
|
How do I change the background color for the item
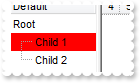
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
long hC = var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->PutItemBackColor(hC,RGB(255,0,0));
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
271
|
How do I expand or collapse an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
270
|
How do I associate an extra data to an item
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemData(var_Items->AddItem("item"),"your extra data");
|
269
|
How do I get the number or count of items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->AddItem(var_Items1->GetItemCount());
|
268
|
How can I specify the width of the ActiveX control, when using the InsertControlItem property
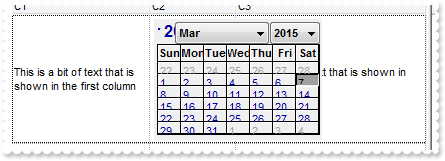
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutScrollBySingleLine(VARIANT_FALSE);
spGantt1->PutTreeColumnIndex(1);
spGantt1->PutDrawGridLines(EXGANTTLib::exAllLines);
spGantt1->GetColumns()->Add(L"C1");
spGantt1->GetColumns()->Add(L"C2");
spGantt1->GetColumns()->Add(L"C3");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->InsertControlItem(vtMissing,L"MSCAL.Calendar",vtMissing);
var_Items->PutItemWidth(h,128);
var_Items->PutCellCaption(h,long(0),"This is a bit of text that is shown in the first column");
var_Items->PutCellSingleLine(h,long(0),EXGANTTLib::exCaptionWordWrap);
var_Items->PutCellCaption(h,long(2),"This is a bit of text that is shown in the third column");
var_Items->PutCellSingleLine(h,long(2),EXGANTTLib::exCaptionWordWrap);
|
267
|
How can I put the ActiveX control in a different column, when using the InsertControlItem property
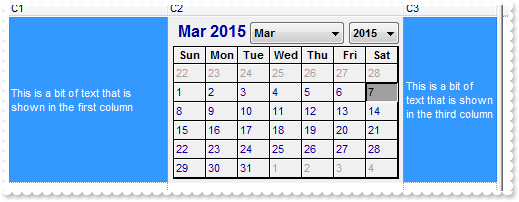
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutScrollBySingleLine(VARIANT_FALSE);
spGantt1->PutDrawGridLines(EXGANTTLib::exAllLines);
spGantt1->GetColumns()->Add(L"C1");
spGantt1->GetColumns()->Add(L"C2");
spGantt1->GetColumns()->Add(L"C3");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->InsertControlItem(vtMissing,L"MSCAL.Calendar",vtMissing);
var_Items->PutCellCaption(h,long(0),"This is a bit of text that is shown in the first column");
var_Items->PutCellSingleLine(h,long(0),EXGANTTLib::exCaptionWordWrap);
var_Items->PutItemWidth(h,-32001);
var_Items->PutCellCaption(h,long(2),"This is a bit of text that is shown in the third column");
var_Items->PutCellSingleLine(h,long(2),EXGANTTLib::exCaptionWordWrap);
|
266
|
Is there any function I can use to get the program or the control identifier I've been using when called the InsertControlItem
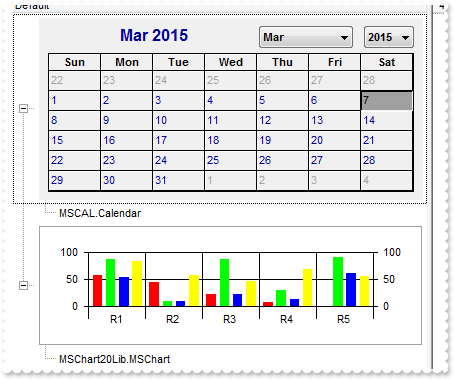
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
spGantt1->PutScrollBySingleLine(VARIANT_FALSE);
spGantt1->PutLinesAtRoot(EXGANTTLib::exLinesAtRoot);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->InsertControlItem(vtMissing,L"MSCAL.Calendar",vtMissing);
var_Items->InsertItem(h,vtMissing,var_Items->GetItemControlID(h));
var_Items->PutExpandItem(h,VARIANT_TRUE);
h = var_Items->InsertControlItem(vtMissing,L"MSChart20Lib.MSChart",vtMissing);
var_Items->PutItemAppearance(h,EXGANTTLib::Etched);
var_Items->InsertItem(h,vtMissing,var_Items->GetItemControlID(h));
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
265
|
How can I change the height of newly created ActiveX control, using the InsertControlItem
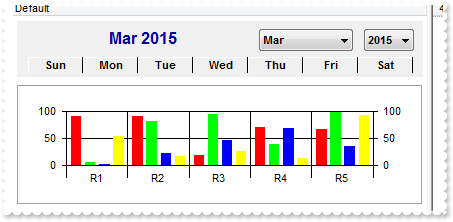
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
spGantt1->PutScrollBySingleLine(VARIANT_FALSE);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemHeight(var_Items->InsertControlItem(vtMissing,L"MSCAL.Calendar",vtMissing),64);
var_Items->PutItemAppearance(var_Items->InsertControlItem(vtMissing,L"MSChart20Lib.MSChart",vtMissing),EXGANTTLib::Etched);
|
264
|
How can I change the border for newly created ActiveX control, using the InsertControlItem
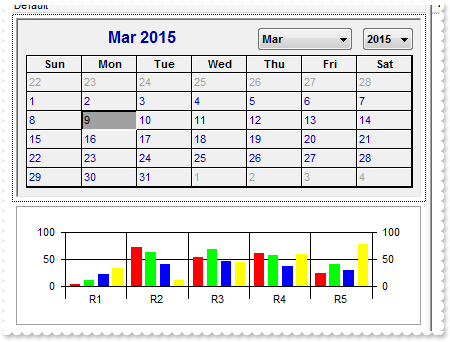
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
spGantt1->PutScrollBySingleLine(VARIANT_FALSE);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutItemAppearance(var_Items->InsertControlItem(vtMissing,L"MSCAL.Calendar",vtMissing),EXGANTTLib::Sunken);
var_Items->PutItemAppearance(var_Items->InsertControlItem(vtMissing,L"MSChart20Lib.MSChart",vtMissing),EXGANTTLib::Etched);
|
263
|
How can I access the properties and methods for an ActiveX control that I've just added using the InsertControlItem
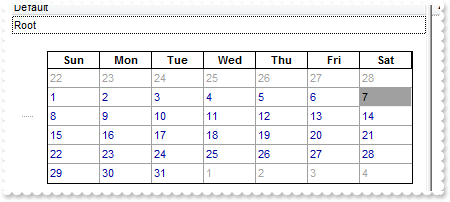
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'MSACAL' for the library: 'Microsoft Calendar Control 2007'
#import <MSCAL.OCX>
*/
MSACAL::ICalendarPtr var_Calendar = ((MSACAL::ICalendarPtr)(var_Items->GetItemObject(var_Items->InsertControlItem(h,L"MSCAL.Calendar",vtMissing))));
var_Calendar->PutBackColor(RGB(255,255,255));
var_Calendar->PutGridCellEffect(0);
var_Calendar->PutShowTitle(VARIANT_FALSE);
var_Calendar->PutShowDateSelectors(VARIANT_FALSE);
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
262
|
How can I access the properties and methods for an ActiveX control that I've just added using the InsertControlItem
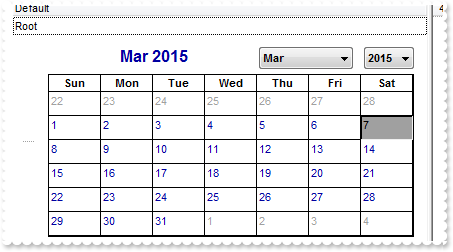
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
/*
Copy and paste the following directives to your header file as
it defines the namespace 'MSACAL' for the library: 'Microsoft Calendar Control 2007'
#import <MSCAL.OCX>
*/
MSACAL::ICalendarPtr var_Calendar = ((MSACAL::ICalendarPtr)(var_Items->GetItemObject(var_Items->InsertControlItem(h,L"MSCAL.Calendar",vtMissing))));
var_Calendar->PutBackColor(RGB(255,255,255));
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
261
|
How can I insert an ActiveX control
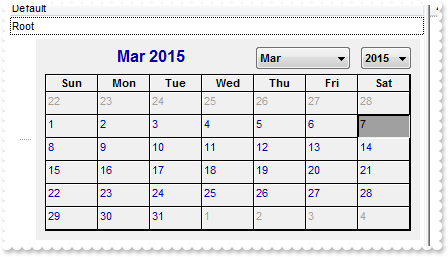
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertControlItem(h,L"MSCAL.Calendar",vtMissing);
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
260
|
How do I programmatically edit a cell

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutAllowEdit(VARIANT_TRUE);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->Edit(var_Items->GetFocusItem(),long(0));
|
259
|
How can I change at runtime the parent of the item
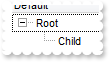
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutLinesAtRoot(EXGANTTLib::exLinesAtRoot);
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long hP = var_Items->AddItem("Root");
long hC = var_Items->AddItem("Child");
var_Items->SetParent(hC,hP);
|
258
|
How can I sort the items
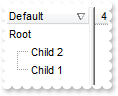
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
spGantt1->GetColumns()->GetItem("Default")->PutSortOrder(EXGANTTLib::SortDescending);
|
257
|
How do I sort the child items

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Root");
var_Items->InsertItem(h,vtMissing,"Child 1");
var_Items->InsertItem(h,vtMissing,"Child 2");
var_Items->PutExpandItem(h,VARIANT_TRUE);
var_Items->SortChildren(h,long(0),VARIANT_FALSE);
|
256
|
How can I ensure or scroll the control so the item fits the control's client area
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
long h = spGantt1->GetItems()->AddItem("item");
spGantt1->GetItems()->EnsureVisibleItem(h);
|
255
|
How can I remove or delete all items
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
spGantt1->GetItems()->AddItem("removed item");
spGantt1->GetItems()->RemoveAllItems();
|
254
|
How can I remove or delete an item
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
long h = spGantt1->GetItems()->AddItem("removed item");
spGantt1->GetItems()->RemoveItem(h);
|
253
|
How can I add or insert child items
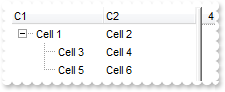
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutLinesAtRoot(EXGANTTLib::exLinesAtRoot);
spGantt1->GetColumns()->Add(L"C1");
spGantt1->GetColumns()->Add(L"C2");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
long h = var_Items->AddItem("Cell 1");
var_Items->PutCellCaption(h,long(1),"Cell 2");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Cell 3"),long(1),"Cell 4");
var_Items->PutCellCaption(var_Items->InsertItem(h,vtMissing,"Cell 5"),long(1),"Cell 6");
var_Items->PutExpandItem(h,VARIANT_TRUE);
|
252
|
How can I add or insert a child item
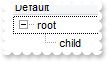
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutLinesAtRoot(EXGANTTLib::exLinesAtRoot);
spGantt1->GetColumns()->Add(L"Default");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->InsertItem(var_Items->AddItem("root"),vtMissing,"child");
|
251
|
How can I add or insert an item
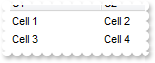
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"C1");
spGantt1->GetColumns()->Add(L"C2");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("Cell 1"),long(1),"Cell 2");
long h = var_Items->AddItem("Cell 3");
var_Items->PutCellCaption(h,long(1),"Cell 4");
|
250
|
How can I add or insert an item

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"Default");
spGantt1->GetItems()->AddItem("new item");
|
249
|
How can I get the columns as they are shown in the control's sortbar
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
ObjectPtr var_Object = ((ObjectPtr)(spGantt1->GetColumns()->GetItemBySortPosition(long(0))));
|
248
|
How can I access the properties of a column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"A");
spGantt1->GetColumns()->GetItem("A")->PutHeaderBold(VARIANT_TRUE);
|
247
|
How can I remove all the columns
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Clear();
|
246
|
How can I remove a column
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Remove("A");
|
245
|
How can I get the number or the count of columns
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
long var_Count = spGantt1->GetColumns()->GetCount();
|
244
|
How can I change the font for all cells in the entire column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'stdole' for the library: 'OLE Automation'
#import <stdole2.tlb>
*/
stdole::FontPtr f = ::CreateObject(L"StdFont");
f->PutName(L"Tahoma");
f->PutSize(_variant_t(long(12)));
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutFont(IFontDispPtr(((stdole::FontPtr)(f))));
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
243
|
How can I change the background color for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutBackColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
242
|
How can I change the foreground color for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutForeColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
241
|
How can I show as strikeout all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutStrikeOut(VARIANT_TRUE);
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
240
|
How can I underline all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutUnderline(VARIANT_TRUE);
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
239
|
How can I show in italic all data in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutItalic(VARIANT_TRUE);
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
238
|
How can I bold the entire column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"1",vtMissing);
var_ConditionalFormat->PutBold(VARIANT_TRUE);
var_ConditionalFormat->PutApplyTo(EXGANTTLib::exFormatToColumns);
spGantt1->GetColumns()->Add(L"Column");
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
237
|
How can I display a computed column and highlight some values that are negative or less than a value
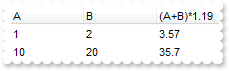
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"A");
spGantt1->GetColumns()->Add(L"B");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"(A+B)*1.19")))->PutComputedField(L"(%0 + %1) * 1.19");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(1)),long(1),long(2));
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaption(var_Items1->AddItem(long(10)),long(1),long(20));
EXGANTTLib::IConditionalFormatPtr var_ConditionalFormat = spGantt1->GetConditionalFormats()->Add(L"%2 > 10",vtMissing);
var_ConditionalFormat->PutBold(VARIANT_TRUE);
var_ConditionalFormat->PutForeColor(RGB(255,0,0));
var_ConditionalFormat->PutApplyTo(EXGANTTLib::FormatApplyToEnum(0x2));
|
236
|
Can I display a computed column so it displays the VAT, or SUM
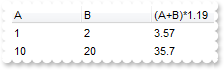
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"A");
spGantt1->GetColumns()->Add(L"B");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"(A+B)*1.19")))->PutComputedField(L"(%0 + %1) * 1.19");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(1)),long(1),long(2));
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaption(var_Items1->AddItem(long(10)),long(1),long(20));
|
235
|
How can I show a column that adds values in the cells
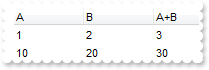
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"A");
spGantt1->GetColumns()->Add(L"B");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"A+B")))->PutComputedField(L"%0 + %1");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(1)),long(1),long(2));
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaption(var_Items1->AddItem(long(10)),long(1),long(20));
|
234
|
Is there any function to filter the control's data as I type, so the items being displayed include the typed characters
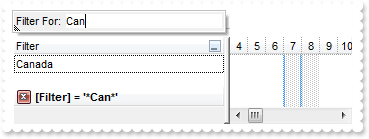
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Filter")));
var_Column->PutFilterOnType(VARIANT_TRUE);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutAutoSearch(EXGANTTLib::exContains);
spGantt1->GetItems()->AddItem("Canada");
spGantt1->GetItems()->AddItem("USA");
|
233
|
Is there any function to filter the control's data as I type, something like filter on type
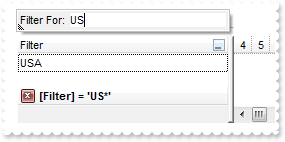
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Filter")));
var_Column->PutFilterOnType(VARIANT_TRUE);
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
spGantt1->GetItems()->AddItem("Canada");
spGantt1->GetItems()->AddItem("USA");
|
232
|
How can I programmatically filter a column
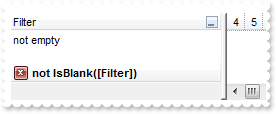
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Filter")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutFilterType(EXGANTTLib::exNonBlanks);
spGantt1->GetItems()->AddItem(vtMissing);
spGantt1->GetItems()->AddItem("not empty");
spGantt1->ApplyFilter();
|
231
|
How can I show or display the control's filter
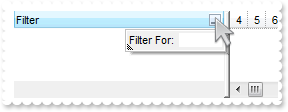
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Filter")))->PutDisplayFilterButton(VARIANT_TRUE);
|
230
|
How can I customize the items being displayed in the drop down filter window
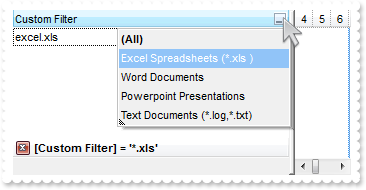
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Custom Filter")));
var_Column->PutDisplayFilterButton(VARIANT_TRUE);
var_Column->PutDisplayFilterPattern(VARIANT_FALSE);
var_Column->PutCustomFilter(_bstr_t("Excel Spreadsheets (*.xls )||*.xls|||Word Documents||*.doc|||Powerpoint Presentations||*.pps|||Text Documents (*.log,*.txt)||*.") +
"txt|*.log");
var_Column->PutFilterType(EXGANTTLib::exPattern);
var_Column->PutFilter(L"*.xls");
spGantt1->GetItems()->AddItem("excel.xls");
spGantt1->GetItems()->AddItem("word.doc");
spGantt1->GetItems()->AddItem("pp.pps");
spGantt1->GetItems()->AddItem("text.txt");
spGantt1->ApplyFilter();
|
229
|
How can I change the order or the position of the columns in the sort bar
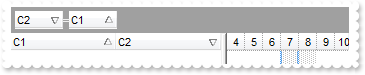
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutSortBarVisible(VARIANT_TRUE);
spGantt1->PutSortBarColumnWidth(48);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"C1")))->PutSortOrder(EXGANTTLib::SortAscending);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"C2")))->PutSortOrder(EXGANTTLib::SortDescending);
spGantt1->GetColumns()->GetItem("C2")->PutSortPosition(0);
|
228
|
How do I arrange my columns on multiple levels

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"S")))->PutWidth(32);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 2")))->PutLevelKey(long(1));
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 3")))->PutLevelKey(long(1));
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 4")))->PutLevelKey(long(1));
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 1")))->PutLevelKey("2");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 2")))->PutLevelKey("2");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 3")))->PutLevelKey("2");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Level 4")))->PutLevelKey("2");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"E")))->PutWidth(32);
|
227
|
How do I arrange my columns on multiple lines
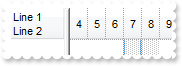
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutHeaderHeight(32);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"")))->PutHTMLCaption(L"Line 1<br>Line 2");
|
226
|
How can I display all cells using HTML format
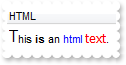
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"HTML")))->PutDef(EXGANTTLib::exCellCaptionFormat,long(1));
spGantt1->GetItems()->AddItem("<font ;12>T</font>his <b>is</b> an <a>html</a> <font Tahoma><fgcolor=FF0000>text</fgcolor></font>.");
|
225
|
How can I display all cells using multiple lines
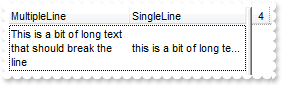
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"MultipleLine")))->PutDef(EXGANTTLib::exCellSingleLine,VARIANT_FALSE);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"SingleLine")))->PutDef(EXGANTTLib::exCellSingleLine,VARIANT_TRUE);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("This is a bit of long text that should break the line"),long(1),"this is a bit of long text that's displayed on a single line");
|
224
|
How do change the vertical alignment for all cells in the column
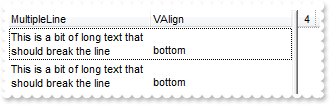
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"MultipleLine")))->PutDef(EXGANTTLib::exCellSingleLine,VARIANT_FALSE);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"VAlign")))->PutDef(EXGANTTLib::exCellVAlignment,long(2));
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem("This is a bit of long text that should break the line"),long(1),"bottom");
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaption(var_Items1->AddItem("This is a bit of long text that should break the line"),long(1),"bottom");
|
223
|
How do change the foreground color for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"ForeColor")))->PutDef(EXGANTTLib::exCellForeColor,long(255));
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
222
|
How do change the background color for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"BackColor")))->PutDef(EXGANTTLib::exCellBackColor,long(255));
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
221
|
How do I show buttons for all cells in the column
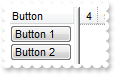
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Button")));
var_Column->PutDef(EXGANTTLib::exCellHasButton,VARIANT_TRUE);
var_Column->PutDef(EXGANTTLib::exCellButtonAutoWidth,VARIANT_TRUE);
spGantt1->GetItems()->AddItem(" Button 1 ");
spGantt1->GetItems()->AddItem(" Button 2 ");
|
220
|
How do I show buttons for all cells in the column
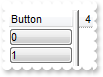
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Button")))->PutDef(EXGANTTLib::exCellHasButton,VARIANT_TRUE);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
219
|
How do I display radio buttons for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Radio")))->PutDef(EXGANTTLib::exCellHasRadioButton,VARIANT_TRUE);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
218
|
How do I display checkboxes for all cells in the column

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Check")))->PutDef(EXGANTTLib::exCellHasCheckBox,VARIANT_TRUE);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
217
|
How can I display a tooltip when the cursor hovers the column
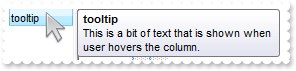
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"tooltip")))->PutToolTip(L"This is a bit of text that is shown when user hovers the column.");
|
216
|
Is there any function to assign a key to a column instead using its name or capion
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Data")))->PutKey(L"DKey");
spGantt1->GetColumns()->GetItem("DKey")->PutCaption(L"new caption");
|
215
|
Is there any function to assign any extra data to a column
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Data")))->PutData("your extra data");
|
214
|
By default, the column gets sorted descending, when I first click its header. How can I change so the column gets sorted ascending when the user first clicks the column's header
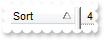
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Sort")))->PutDefaultSortOrder(VARIANT_TRUE);
|
213
|
How can I specify the maximum width for the column, if I use WidthAutoResize property

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Auto")));
var_Column->PutWidthAutoResize(VARIANT_TRUE);
var_Column->PutMinWidthAutoResize(32);
var_Column->PutMaxWidthAutoResize(128);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
212
|
How can I specify the minimum width for the column, if I use WidthAutoResize property

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Auto")));
var_Column->PutWidthAutoResize(VARIANT_TRUE);
var_Column->PutMinWidthAutoResize(32);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
211
|
Is there any option to resize the column based on its data, captions

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"A")))->PutWidthAutoResize(VARIANT_TRUE);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
210
|
How can I align the icon in the column's header in the center
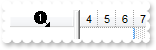
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"")));
var_Column->PutHeaderImage(1);
var_Column->PutHeaderImageAlignment(EXGANTTLib::CenterAlignment);
|
209
|
How do I align the icon in the column's header to the right

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->Images(_bstr_t("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq") +
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" +
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" +
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=");
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"ColumnName")));
var_Column->PutHeaderImage(1);
var_Column->PutHeaderImageAlignment(EXGANTTLib::RightAlignment);
|
208
|
How do I show or hide the sorting icons, but still need sorting
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Sorted")))->PutSortOrder(EXGANTTLib::SortAscending);
spGantt1->GetColumns()->GetItem(long(0))->PutDisplaySortIcon(VARIANT_FALSE);
|
207
|
How do I enable or disable the entire column
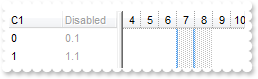
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->GetColumns()->Add(L"C1");
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Disabled")))->PutEnabled(VARIANT_FALSE);
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaption(var_Items->AddItem(long(0)),long(1),"0.1");
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaption(var_Items1->AddItem(long(1)),long(1),"1.1");
|
206
|
How do I disable drag and drop columns
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"C1")))->PutAllowDragging(VARIANT_FALSE);
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"C2")))->PutAllowDragging(VARIANT_FALSE);
|
205
|
How do I disable resizing a column at runtime
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Unsizable")))->PutAllowSizing(VARIANT_FALSE);
spGantt1->GetColumns()->Add(L"C2");
spGantt1->GetColumns()->Add(L"C3");
spGantt1->GetColumns()->Add(L"C4");
|
204
|
How can I align the column to the right, and its caption too
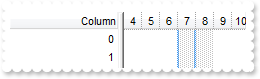
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
EXGANTTLib::IColumnPtr var_Column = ((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Column")));
var_Column->PutAlignment(EXGANTTLib::RightAlignment);
var_Column->PutHeaderAlignment(EXGANTTLib::RightAlignment);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
203
|
How can I align the column to the right
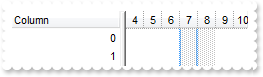
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Column")))->PutAlignment(EXGANTTLib::RightAlignment);
spGantt1->GetItems()->AddItem(long(0));
spGantt1->GetItems()->AddItem(long(1));
|
202
|
How do I change the column's caption

/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
((EXGANTTLib::IColumnPtr)(spGantt1->GetColumns()->Add(L"Column")))->PutCaption(L"new caption");
|
201
|
Can I change the visual effect, appearance for the anchor, hyperlink elements, in HTML captions, after the user clicks it
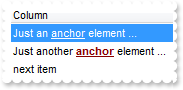
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXGANTTLib' for the library: 'ExGantt 1.0 Control Library'
#import <ExGantt.dll>
using namespace EXGANTTLib;
*/
EXGANTTLib::IGanttPtr spGantt1 = GetDlgItem(IDC_GANTT1)->GetControlUnknown();
spGantt1->PutFormatAnchor(VARIANT_FALSE,L"<b><u><fgcolor=880000> </fgcolor></u></b>");
spGantt1->GetColumns()->Add(L"Column");
EXGANTTLib::IItemsPtr var_Items = spGantt1->GetItems();
var_Items->PutCellCaptionFormat(var_Items->AddItem("Just an <a1>anchor</a> element ..."),long(0),EXGANTTLib::exHTML);
EXGANTTLib::IItemsPtr var_Items1 = spGantt1->GetItems();
var_Items1->PutCellCaptionFormat(var_Items1->AddItem("Just another <a2>anchor</a> element ..."),long(0),EXGANTTLib::exHTML);
spGantt1->GetItems()->AddItem("next item");
|